You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Describe the bug
Running a Vue Test Utils unit test with the VueTour package included produces a memory leak. When logging heap usage or detecting memory leaks in Jest, the memory usage can be seen increasing with every test, which only occurs when the VueTour package is included.
Take for example this following very basic vue component: Memory.vue
import { mount } from "@vue/test-utils";
import MemoryTest from "../components/MemoryTest.vue";
import { createLocalVue } from "@vue/test-utils";
const localVue = createLocalVue();
describe("MemoryTest", () => {
let wrapper = null;
beforeEach(() => {
wrapper = mount(MemoryTest, { localVue });
});
afterEach(() => {
wrapper.destroy();
});
it("performs a test", () => {
expect(wrapper.text()).toContain("memory test");
});
});
If I run this test with the following options node ./node_modules/jest/bin/jest.js --runInBand --logHeapUsage --silent --detect-leaks
The test passes successfully:
However, if the unit test file is edited to include the VueTour library, and the same test is ran, the --detect-leaks option will fail due to the test now leaking memory: Updated Memory.test.js file with issue present:
import { mount } from "@vue/test-utils";
import MemoryTest from "../components/MemoryTest.vue";
import VueTour from "vue-tour";
import { createLocalVue } from "@vue/test-utils";
const localVue = createLocalVue();
localVue.use(VueTour);
describe("MemoryTest", () => {
let wrapper = null;
beforeEach(() => {
wrapper = mount(MemoryTest, { localVue });
});
afterEach(() => {
wrapper.destroy();
});
it("performs a test", () => {
expect(wrapper.text()).toContain("memory test");
});
});
To Reproduce
Steps to reproduce the behavior:
Create a new Vue project with the Memory.vue and Memory.test.js files described above (Jest and Vue Test Utils must be included in the project)
Run the unit test using the following command node ./node_modules/jest/bin/jest.js --runInBand --logHeapUsage --silent --detect-leaks
See the error in the console about a detected memory leak
Expected behaviour
The tests should run without increasing the heap size every test. Or when the '--detect-leaks' option is used the test should pass instead of failing due to a memory leak.
Screenshots
If you don't use the --detect-leaks option, the tests will pass but you will clearly be able to see increased memory usage with each test that passes. In the following screenshot, I included the VueTour package in jest_setup.js so that it would be used in every test, to show off the memory leak:
Desktop (please complete the following information):
OS: Windows
Browser: n/a
vue-tour Version: 2.0.0
Jest Version: 26.6.3
@vue/test-utils Version: 1.3.0
Additional context
The 'weak-napi' NPM package must be installed to use the '--detect-leaks' option in Jest.
The text was updated successfully, but these errors were encountered:
Describe the bug
Running a Vue Test Utils unit test with the VueTour package included produces a memory leak. When logging heap usage or detecting memory leaks in Jest, the memory usage can be seen increasing with every test, which only occurs when the VueTour package is included.
Take for example this following very basic vue component:
Memory.vue
And create a unit test for it:
Memory.test.js
If I run this test with the following options
node ./node_modules/jest/bin/jest.js --runInBand --logHeapUsage --silent --detect-leaks
The test passes successfully:
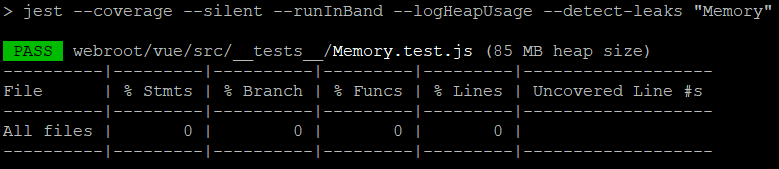
However, if the unit test file is edited to include the VueTour library, and the same test is ran, the --detect-leaks option will fail due to the test now leaking memory:
Updated Memory.test.js file with issue present:
To Reproduce
Steps to reproduce the behavior:
node ./node_modules/jest/bin/jest.js --runInBand --logHeapUsage --silent --detect-leaks
Expected behaviour
The tests should run without increasing the heap size every test. Or when the '--detect-leaks' option is used the test should pass instead of failing due to a memory leak.
Screenshots
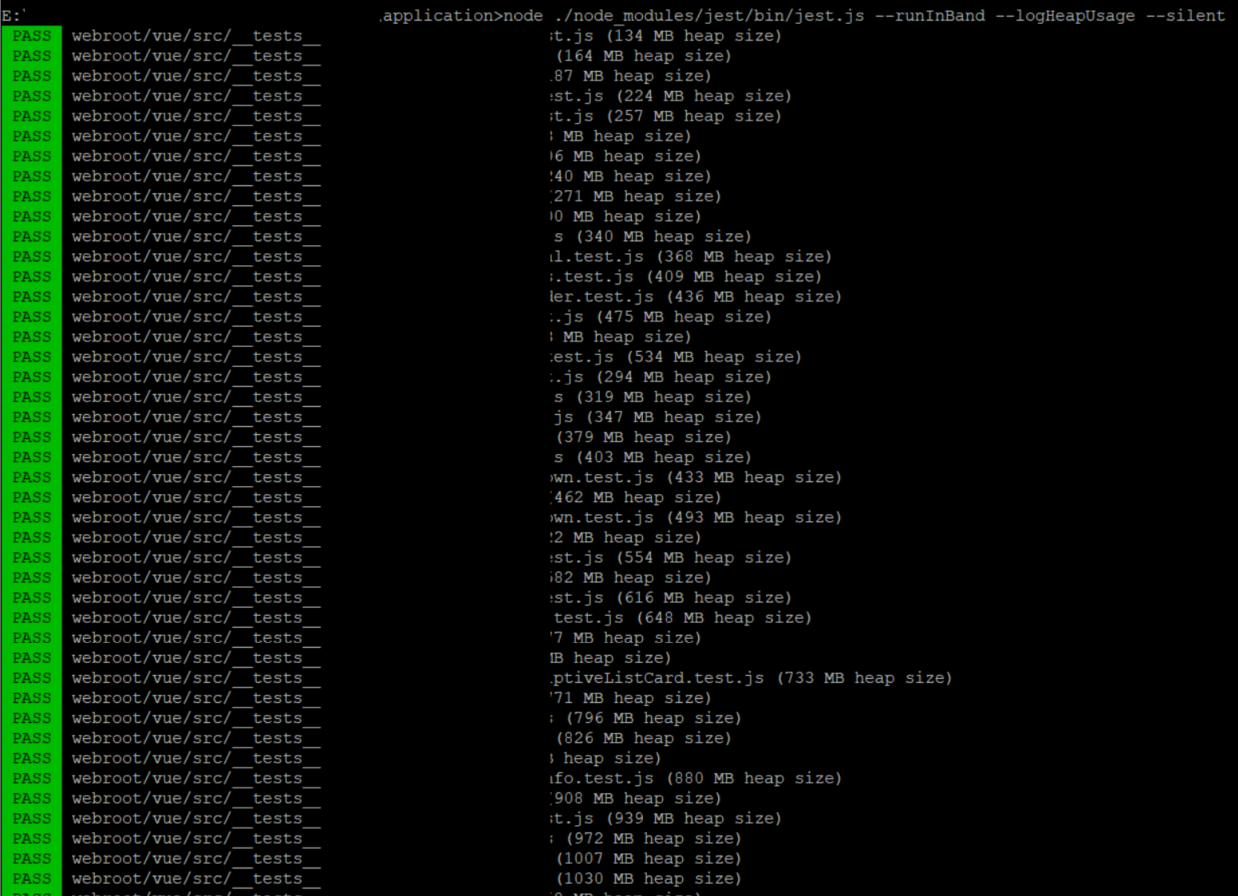
If you don't use the --detect-leaks option, the tests will pass but you will clearly be able to see increased memory usage with each test that passes. In the following screenshot, I included the VueTour package in jest_setup.js so that it would be used in every test, to show off the memory leak:
Desktop (please complete the following information):
Additional context
The 'weak-napi' NPM package must be installed to use the '--detect-leaks' option in Jest.
The text was updated successfully, but these errors were encountered: