This is simple maker for welcome and leave cards in discord bot in discord.py or pycord.
Python 3.8 or higher is required
# Linux/macOS
pip3 install -U maxi-card.py
# Windows
pip install -U maxi-card.py
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.event
async def on_member_join(member):
#guild definition
guild = member.guild
#welcome channel definition (id=YourWelcomeChannelID)
channel = discord.utils.get(guild.text_channels, id=753239660230082690)
#creating welcome card object
card = WelcomeCard()
#setting member name
card.member = member
#setting account created time
card.datetime = member.created_at.strftime("%d, %B %Y, %H:%M %p")
#setting server
card.server = guild
#sending image to discord channel
await channel.send(file=await card.create())
client.run("TOKEN")
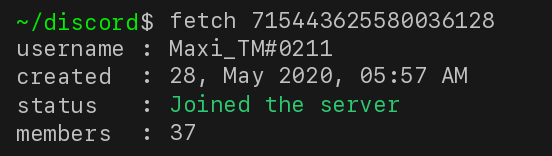
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.event
async def on_member_remove(member):
#guild definition
guild = member.guild
#welcome channel definition (id=YourLeaveChannelID)
channel = discord.utils.get(guild.text_channels, id=753239660230082690)
#creating leave card object
card = LeaveCard()
#setting member name
card.member = member
#setting account created time
card.datetime = member.created_at.strftime("%d, %B %Y, %H:%M %p")
#setting server
card.server = guild
#sending image to discord channel
await channel.send(file=await card.create())
client.run("TOKEN")
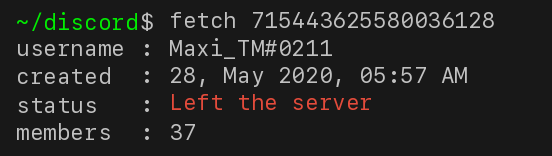
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def wanted(ctx):
#creating wanted card object
card = WantedCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
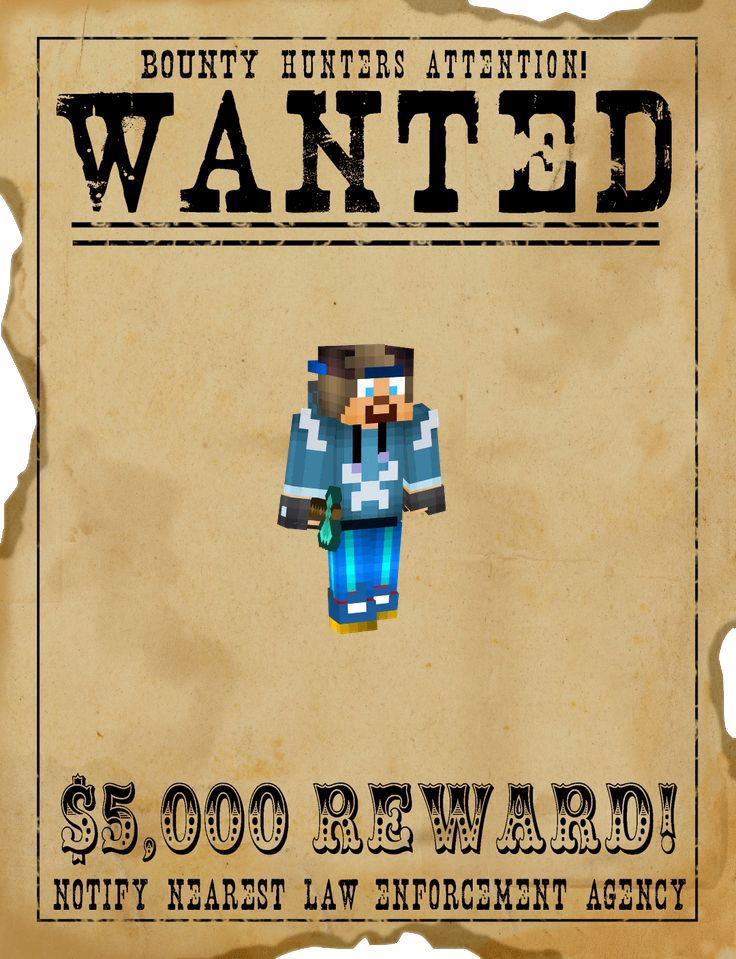
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def delete(ctx):
#creating delete card object
card = DeleteCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
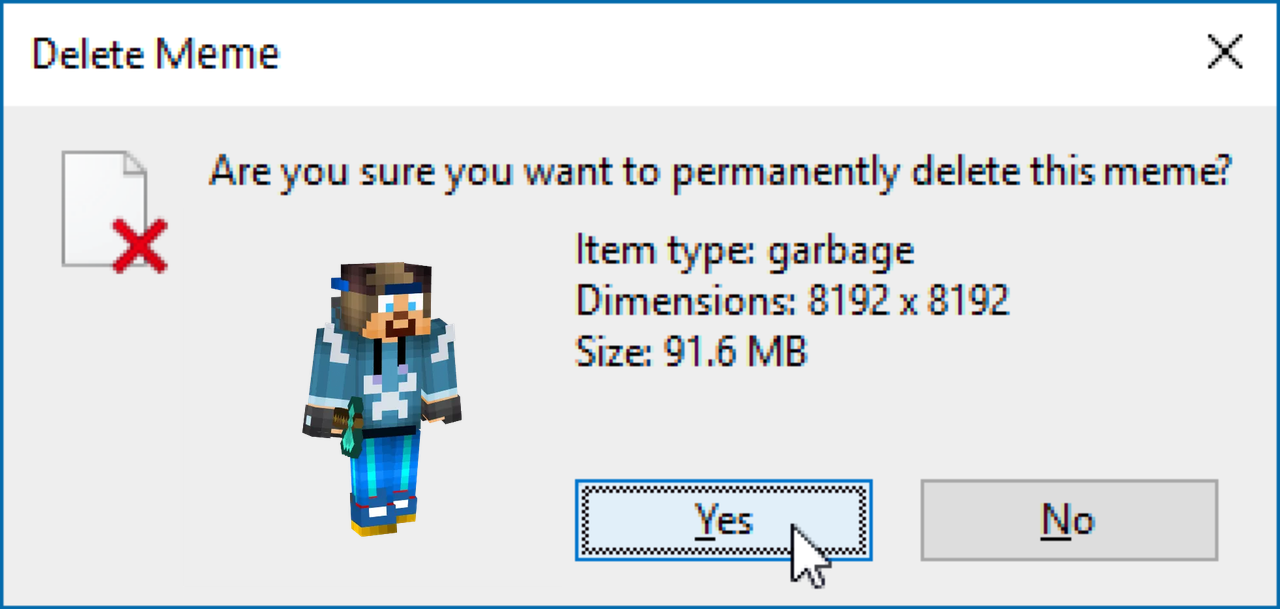
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def trash(ctx):
#creating trash card object
card = TrashCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
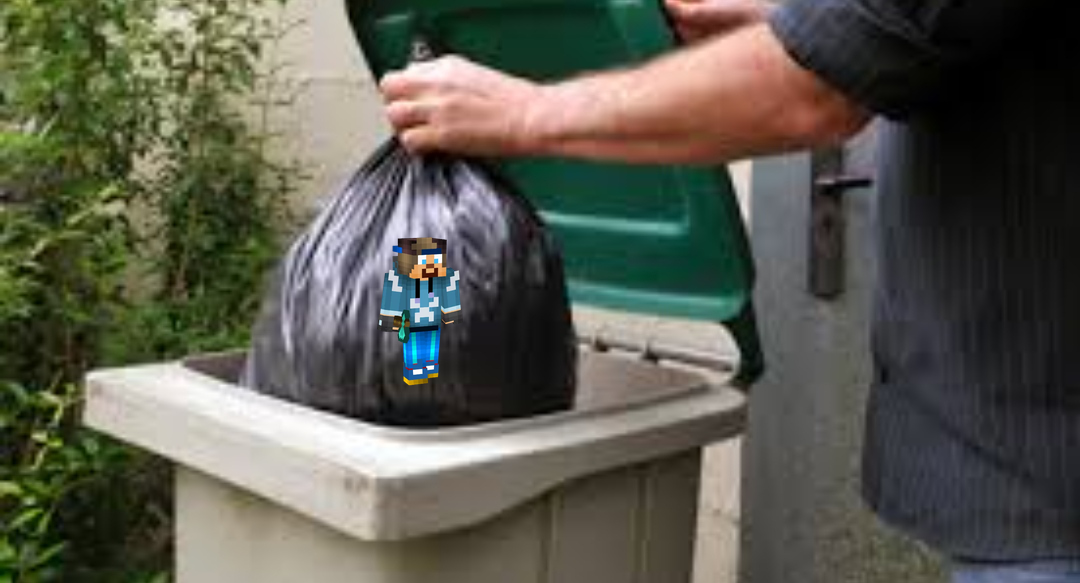
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def tombstone(ctx):
#creating tombstone card object
card = TombstoneCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
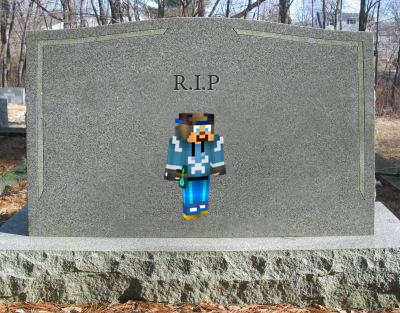
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def hitler(ctx):
#creating hitler card object
card = HitlerCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
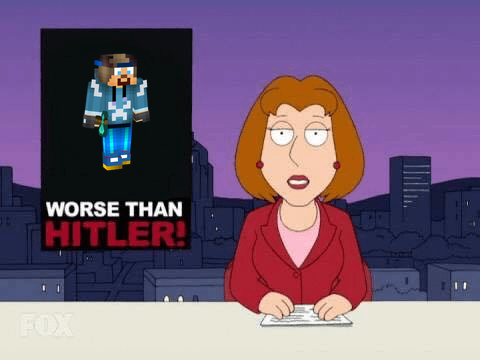
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def jail(ctx):
#creating jail card object
card = JailCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
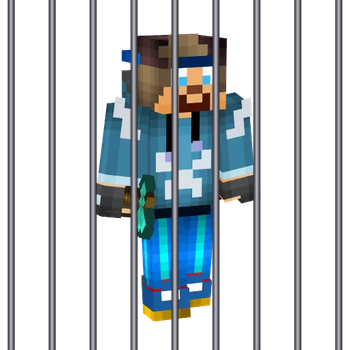
import discord
from discord.ext import commands
from maxicard import *
intents = discord.Intents.default()
intents.members = True
client = commands.Bot(command_prefix="!", intents=intents)
@client.command()
async def missionpassed(ctx):
#creating passed card object
card = PassedCard()
#setting avatar image
card.avatar = ctx.author.avatar_url
#sending image to discord channel
await ctx.send(file=await card.create())
client.run("TOKEN")
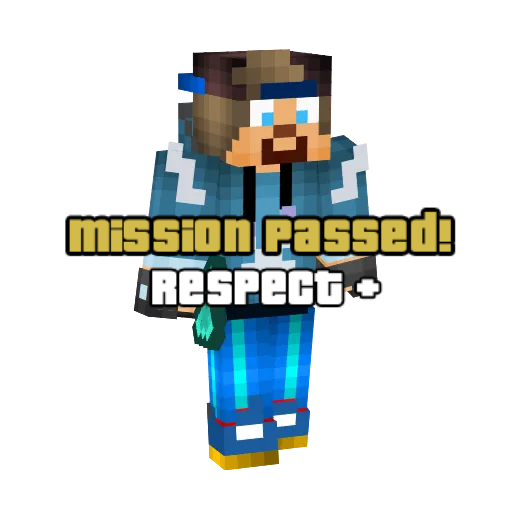