-
-
Notifications
You must be signed in to change notification settings - Fork 2.9k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
docs: added plugins documentation (#10989)
(Should be merged after the next release) Closes DX-1294
- Loading branch information
1 parent
0c5f21e
commit c26ef84
Showing
26 changed files
with
2,901 additions
and
52 deletions.
There are no files selected for viewing
17 changes: 17 additions & 0 deletions
17
www/apps/book/app/learn/customization/reuse-customizations/page.mdx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
export const metadata = { | ||
title: `${pageNumber} Re-Use Customizations with Plugins`, | ||
} | ||
|
||
# {metadata.title} | ||
|
||
In the previous chapters, you've learned important concepts related to creating modules, implementing commerce features in workflows, exposing those features in API routes, customizing the Medusa Admin dashboard with Admin Extensions, and integrating third-party systems. | ||
|
||
You've implemented the brands example within a single Medusa application. However, this approach is not scalable when you want to reuse your customizations across multiple projects. | ||
|
||
To reuse your customizations across multiple Medusa applications, such as implementing brands in different projects, you can create a plugin. A plugin is an NPM package that encapsulates your customizations and can be installed in any Medusa application. Plugins can include modules, workflows, API routes, Admin Extensions, and more. | ||
|
||
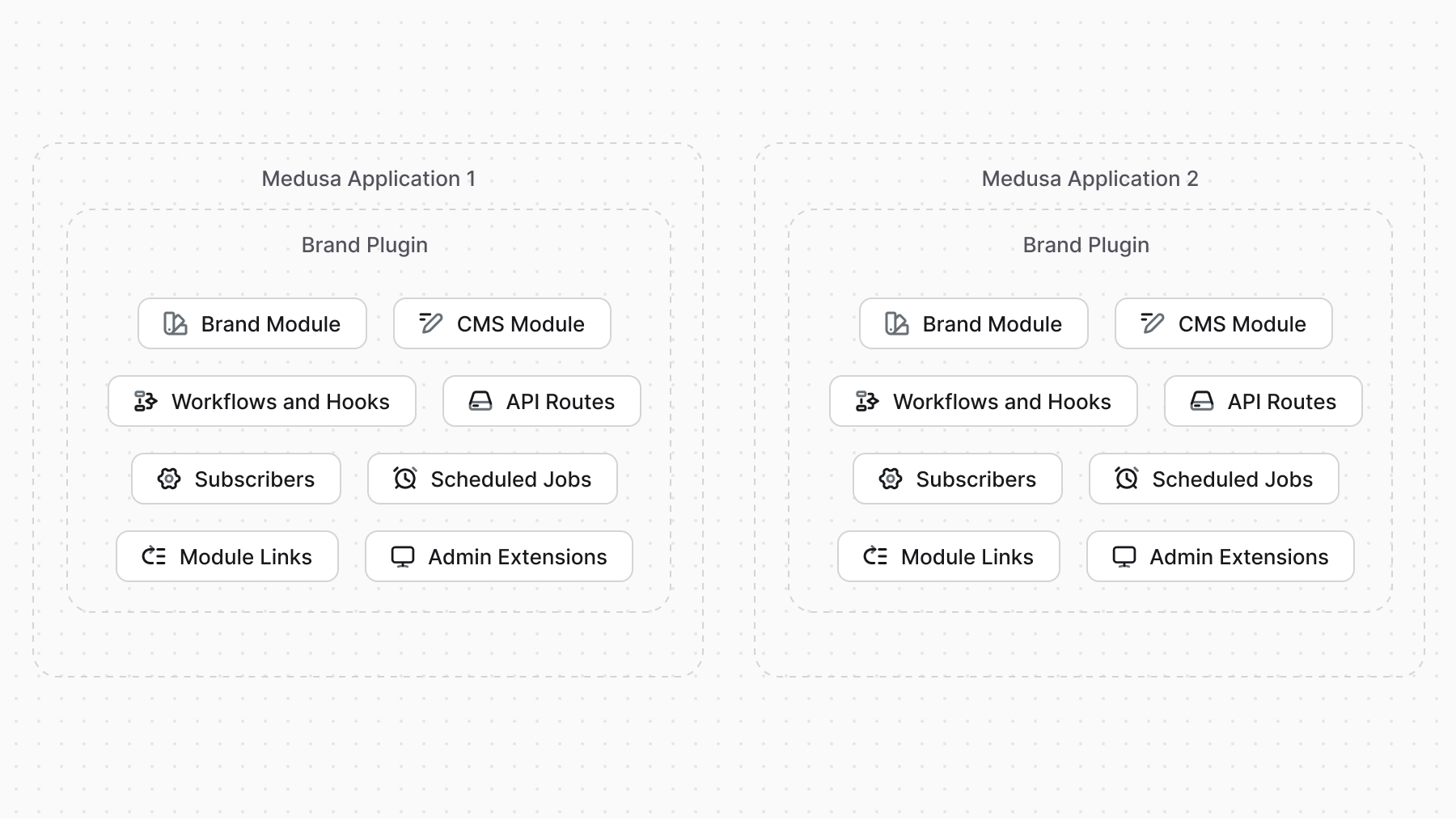 | ||
|
||
Medusa provides the tooling to create a plugin package, test it in a local Medusa application, and publish it to NPM. | ||
|
||
To learn more about plugins and how to create them, refer to [this chapter](../../fundamentals/plugins/page.mdx). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.