This repository has been archived by the owner on Nov 16, 2023. It is now read-only.
-
Notifications
You must be signed in to change notification settings - Fork 50
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
fix: avoid extra padding in screenshots on devices that use row-padde…
…d images (#20) #### Description of changes This fixes the issue described by #19 by detecting the case where the system has provided an image data with row padding and creating an intermediate, unpadded image buffer before invoking `Bitmap::copyPixelsFromBuffer`. I explored a few other implementation options in the hopes of avoiding having to allocate the intermediate buffer, but they all came up short because `copyPixelsFromBuffer` is the only method `Bitmap` exposes that copies the backing data *exactly as-is* from the original source data, without applying any color transformations on the data. In particular, the variants of `Bitmap::createBitmap` and `Bitmap::setPixels` that accept `int[] colors` parameters look promising because some of them have built-in support for explicit `stride` parameters, but all of them perform color transformations (with or without `Bitmap::setPremultiplied`) that result in screenshots with incorrect colors. Besides unit tests, will verify before merging against: * [x] A device that exhibited the bad padding behavior before the fix (my Moto G7 Power) * [x] An emulator that did not previously exhibit the bad padding behavior **Before (note the extra padding at the right side of the screenshot image and the misaligned failure highlight):** 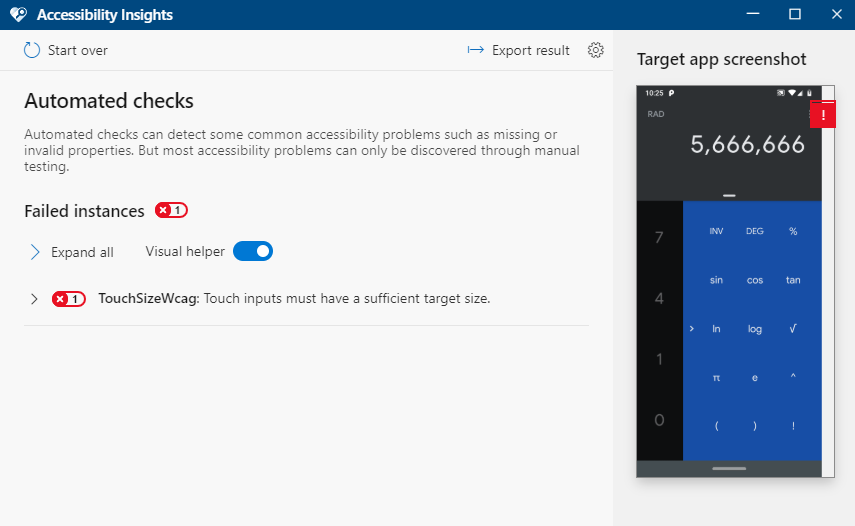 **After (note padding is gone and highlight lines up):** 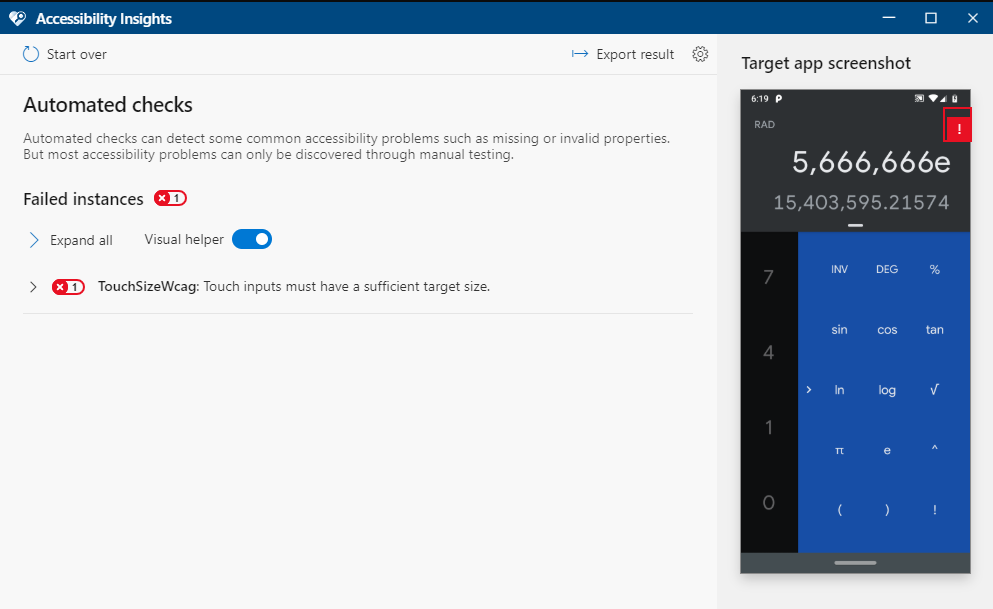 #### Pull request checklist <!-- If a checklist item is not applicable to this change, write "n/a" in the checkbox --> - [x] Addresses an existing issue: #19 - [x] Added/updated relevant unit test(s) - [x] Ran `./gradlew fastpass` from `AccessibilityInsightsForAndroidService` - [x] PR title _AND_ final merge commit title both start with a semantic tag (`fix:`, `chore:`, `feat(feature-name):`, `refactor:`).
- Loading branch information
Showing
3 changed files
with
165 additions
and
33 deletions.
There are no files selected for viewing
10 changes: 10 additions & 0 deletions
10
.../main/java/com/microsoft/accessibilityinsightsforandroidservice/ImageFormatException.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
// Copyright (c) Microsoft Corporation. | ||
// Licensed under the MIT License. | ||
|
||
package com.microsoft.accessibilityinsightsforandroidservice; | ||
|
||
class ImageFormatException extends Exception { | ||
public ImageFormatException(String message) { | ||
super(message); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters