-
Notifications
You must be signed in to change notification settings - Fork 14
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add support for Vue3 plugin & update CLI
In order to support Vue3 we had to drop `vue-template-compiler` in favor of `@vue/compiler-sfc` for compatibility reasons. This way we can now support both Vue2 and Vue3. Side effects `vue/compiler-sfc` ast result is more cryptic than the previous dependency, so we might need to revisit in the future and use an alternative.
- Loading branch information
Showing
17 changed files
with
627 additions
and
18 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
{ | ||
"extends": ["airbnb-base"], | ||
"rules": { | ||
"no-underscore-dangle": "off" | ||
}, | ||
"ignorePatterns": ["dist/"] | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,4 @@ | ||
tests | ||
.eslintrc.yml | ||
coverage | ||
tags |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,205 @@ | ||
<p align="center"> | ||
<a href="https://www.transifex.com"> | ||
<img src="https://raw.githubusercontent.com/transifex/transifex-javascript/master/media/transifex.png" height="60"> | ||
</a> | ||
</p> | ||
<p align="center"> | ||
<i>Transifex Native is a full end-to-end, cloud-based localization stack for moderns apps.</i> | ||
</p> | ||
<p align="center"> | ||
<img src="https://github.com/transifex/transifex-javascript/actions/workflows/npm-publish.yml/badge.svg"> | ||
<a href="https://www.npmjs.com/package/@transifex/vue3"> | ||
<img src="https://img.shields.io/npm/v/@transifex/vue3.svg"> | ||
</a> | ||
<a href="https://developers.transifex.com/docs/native"> | ||
<img src="https://img.shields.io/badge/docs-transifex.com-blue"> | ||
</a> | ||
</p> | ||
|
||
# Transifex Native SDK: Vue i18n | ||
|
||
Vue3 component for localizing Vue application using | ||
[Transifex Native](https://www.transifex.com/native/). | ||
|
||
Related packages: | ||
- [@transifex/native](https://www.npmjs.com/package/@transifex/native) | ||
- [@transifex/cli](https://www.npmjs.com/package/@transifex/cli) | ||
|
||
Learn more about Transifex Native in the [Transifex Developer Hub](https://developers.transifex.com/docs/native). | ||
|
||
# How it works | ||
|
||
**Step1**: Create a Transifex Native project in [Transifex](https://www.transifex.com). | ||
|
||
**Step2**: Grab credentials. | ||
|
||
**Step3**: Internationalize the code using the SDK. | ||
|
||
**Step4**: Push source phrases using the `@transifex/cli` tool. | ||
|
||
**Step5**: Translate the app using over-the-air updates. | ||
|
||
No translation files required. | ||
|
||
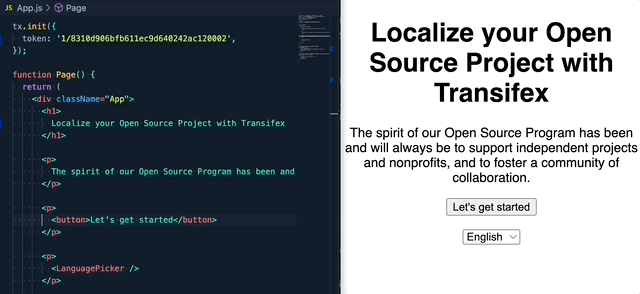 | ||
|
||
# Upgrade to v2 | ||
|
||
If you are upgrading from the `1.x.x` version, please read this [migration guide](https://github.com/transifex/transifex-javascript/blob/HEAD/UPGRADE_TO_V2.md), as there are breaking changes in place. | ||
|
||
|
||
# Install | ||
|
||
Install the library and its dependencies using: | ||
|
||
```sh | ||
npm install @transifex/native @transifex/vue3 --save | ||
``` | ||
|
||
# Usage | ||
|
||
## Initiate the plugin in a Vue App | ||
|
||
```javascript | ||
import { createApp } from 'vue'; | ||
import App from './App.vue'; | ||
import { tx } from '@transifex/native'; | ||
import { TransifexVue } from '@transifex/vue3'; | ||
|
||
tx.init({ | ||
token: '<token>', | ||
}); | ||
|
||
const app = createApp(App); | ||
|
||
app.use(TransifexVue); | ||
app.mount('#app'); | ||
|
||
``` | ||
|
||
|
||
## `T` Component | ||
|
||
```javascript | ||
<template> | ||
<div> | ||
<T _str="Hello world" /> | ||
<T _str="Hello {username}" :username="user" /> | ||
</div> | ||
</template> | ||
|
||
<script> | ||
export default { | ||
name: 'App', | ||
data() { | ||
return { | ||
user: 'John' | ||
}; | ||
}, | ||
} | ||
</script> | ||
``` | ||
|
||
Available optional props: | ||
|
||
| Prop | Type | Description | | ||
|------------|--------|---------------------------------------------| | ||
| _context | String | String context, affects key generation | | ||
| _key | String | Custom string key | | ||
| _comment | String | Developer comment | | ||
| _charlimit | Number | Character limit instruction for translators | | ||
| _tags | String | Comma separated list of tags | | ||
|
||
|
||
## `UT` Component | ||
|
||
```javascript | ||
<template> | ||
<div> | ||
<UT _str="Hello <b>{username}</b>" :username="user" /> | ||
<p> | ||
<UT _str="Hello <b>{username}</b>" :username="user" _inline="true" /> | ||
</p> | ||
</div> | ||
</template> | ||
|
||
<script> | ||
export default { | ||
name: 'App', | ||
data() { | ||
return { | ||
user: 'John' | ||
}; | ||
}, | ||
} | ||
</script> | ||
``` | ||
|
||
`UT` has the same behaviour as `T`, but renders source string as HTML inside a | ||
`div` tag. | ||
|
||
Available optional props: All the options of `T` plus: | ||
|
||
| Prop | Type | Description | | ||
|---------|---------|-------------------------------------------------| | ||
| _inline | Boolean | Wrap translation in `span` | | ||
|
||
## `$t` template function or `this.t` alias for scripts | ||
|
||
Makes the current component re-render when a language change is detected and | ||
returns a t-function you can use to translate strings programmatically. | ||
|
||
You will most likely prefer to use the `T` or `UT` components over this, unless | ||
for some reason you want to have the translation output in a variable for | ||
manipulation. | ||
|
||
```javascript | ||
<template> | ||
<div> | ||
{{$t('Hello world').toLowerCase()}} | ||
{{hellofromscript}} | ||
</div> | ||
</template> | ||
|
||
<script> | ||
export default { | ||
name: 'App', | ||
computed: { | ||
hellofromscript: function() { return this.t('Hello world').toLowerCase() }, | ||
}, | ||
} | ||
</script> | ||
|
||
``` | ||
|
||
## `LanguagePicker` component | ||
|
||
Renders a `<select>` tag that displays supported languages and switches the | ||
application's selected language on change. | ||
|
||
```javascript | ||
<template> | ||
<div> | ||
<T _str="This is a translatable message" /> | ||
<LanguagePicker /> | ||
</div> | ||
</template> | ||
|
||
<script> | ||
import { LanguagePicker } from '@transifex/vue3'; | ||
export default { | ||
name: 'App', | ||
components: { | ||
LanguagePicker, | ||
} | ||
} | ||
</script> | ||
``` | ||
|
||
Accepts properties: | ||
|
||
- `className`: The CSS class that will be applied to the `<select>` tag. | ||
|
||
# License | ||
|
||
Licensed under Apache License 2.0, see [LICENSE](https://github.com/transifex/transifex-javascript/blob/HEAD/LICENSE) file. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
module.exports = { | ||
presets: [['@babel/preset-env', { targets: { node: 'current' } }]], | ||
}; |
Oops, something went wrong.